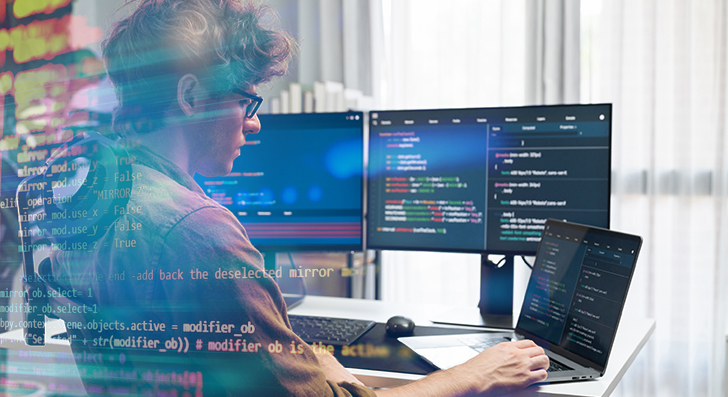
Scalability means your application can deal with growth—extra people, far more information, and much more traffic—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect within your prepare from the start. A lot of applications fall short once they improve quickly for the reason that the first style and design can’t deal with the additional load. As a developer, you must think early about how your procedure will behave under pressure.
Start off by developing your architecture to generally be flexible. Steer clear of monolithic codebases exactly where anything is tightly connected. In its place, use modular design and style or microservices. These styles split your application into smaller sized, unbiased parts. Every single module or service can scale on its own with no influencing The full system.
Also, take into consideration your databases from day one particular. Will it need to manage one million end users or just 100? Pick the correct sort—relational or NoSQL—based on how your facts will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
A different vital point is to prevent hardcoding assumptions. Don’t publish code that only will work less than current situations. Contemplate what would take place In the event your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like message queues or event-driven techniques. These support your application take care of additional requests devoid of finding overloaded.
Any time you Make with scalability in your mind, you are not just planning for achievement—you are lowering long term headaches. A well-prepared process is simpler to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Selecting the right databases can be a important Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe result in failures as your app grows.
Start by being familiar with your knowledge. Is it really structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are generally powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and facts.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing huge volumes of unstructured or semi-structured details and may scale horizontally extra effortlessly.
Also, look at your read and publish styles. Have you been accomplishing a lot of reads with less writes? Use caching and read replicas. Will you be managing a hefty publish load? Look into databases that will take care of superior create throughput, as well as celebration-primarily based knowledge storage units like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need Superior scaling characteristics now, but picking a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain patterns. And often check database efficiency while you expand.
In a nutshell, the best databases will depend on your application’s composition, pace wants, And the way you assume it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is essential to scalability. As your application grows, just about every modest delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s vital that you Develop successful logic from the start.
Commence by creating thoroughly clean, easy code. Steer clear of repeating logic and take away just about anything unwanted. Don’t select the most sophisticated solution if a simple 1 works. Keep the capabilities quick, focused, and simple to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes far too extended to operate or employs an excessive amount of memory.
Future, have a look at your database queries. These generally slow matters down over the code alone. Ensure Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and as an alternative select distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Primarily across massive tables.
If you recognize a similar information staying asked for repeatedly, use caching. Retail outlet the results temporarily employing resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to check with massive datasets. Code and queries that do the job fine with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and more targeted traffic. If anything goes by just one server, it can immediately turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming visitors across multiple servers. In lieu of a person server executing the many operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server will get overloaded. If 1 server goes down, the load balancer can send traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When consumers request the same facts once again—like an item web page or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for quick obtain.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. With each other, they assist your application handle far more buyers, stay speedy, and Recuperate from troubles. If you propose to develop, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess future capacity. When visitors will increase, you can add much more sources with only a few clicks or immediately making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to creating your app instead of handling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a more info laptop computer for the cloud, with no surprises. Docker is the most popular tool for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into solutions. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, using cloud and container equipment means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, start out utilizing these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app way too. Control just how long it will require for people to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, it is best to get notified promptly. This will help you correct concerns quick, frequently before buyers even see.
Checking is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, targeted traffic and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Ultimate Thoughts
Scalability isn’t only for large corporations. Even little applications require a robust Basis. By developing carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make apps that increase effortlessly without having breaking stressed. Begin modest, Believe massive, and Establish intelligent.